Laravel 9 - How to Add Toastr Notifications
Hello Artisans, today I'll talk about implementing Toastr notifications in our Laravel application. Toastr is a JavaScript library that displays the message in the form of a notification. It can be a success, warning, info, or error. It is necessary when a user performs an action and we've to notify that user. So, let's see how we can use Toastr notifications in our application.
Note: Tested on Laravel 9.2.
- Install brian2694/laravel-toastr
- Add CSS and JS to html
- Create and Setup Controller
- Define Route
- Output
Install brian2694/laravel-toastr
Firstly we need to install the brian2694/laravel-toastr package. Run the below command in your terminal to install :
Add CSS and JS to html
Now we will modify the Laravel default welcome.blade.php. So, put the below code in yours.
Create and Setup Controller
First we'll create a controller where we can use our logic to show user a toastr notification. Fire the below command in your terminal to create a controller.
Now put the below code in ToastrNotificationController.php.
Define Route
Now Replace your web.php file with the below codes.
Output
Now if you go to http://127.0.0.1:8000/toastr-notification you'll see the below notification in the top right-most corner.
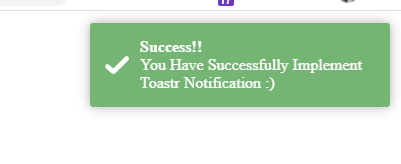